In this post I’ll show you some code on how to generate a star rating for bootstrap. Up to now, I was enjoying the free version of MDBootstrap 4.x for quite a while. Therefore I spend some decent effort on migrating one of my medium sized projects to the new released 5.0 alpha-2. The free version should have enough functions for use my use cases, or so I thought.
For a product description I wanted to create a 1 to 5 star rating dynamically in html
(as known from pages like amazon). In the past, this component was a plugin in the free 4.x version of MDBootstrap. However, it is now only available in the Pro version. Unfortunately the pro version is *drums rolled* only available as a monthly / yearly abonnement plan. So I decided to switch to a 100% bootstrap version of my project, which btw I can highly recommend. Obviously a 5.0 alpha version is also available for bootstrap, as mdbootstrap is an expansion (= material design for bootstrap). However, generating a star rating in bootstrap is not a native function in vanilla bootstrap.
HTML Requirements
As this functions uses the star icon, you need to import an icon font into your project. To do so, just add the following line between your html <head>
and </head>
tags.
<head>
...
<link rel="stylesheet" href="https://use.fontawesome.com/releases/v5.11.2/css/all.css" />
...
</head>
From now on, you can insert icons in your web page, for more details just have a look at the FontAwesome page.
Star Rating for Bootstrap as JavaScript Function
As I needed this function as a visualization of a products amazon rating, I had to give 1/2 stars not form 0.5 on, but from 0.3 on. Amazon visualizes e.g. a 3.3 rating as 3 full and 1/2 star. Here is the function you can add to one of your .js
files on case you already have one.
function generate_star_rating(rating_value){
// divide the given rating into integer and float part
let int_part = rating_value | 0;
let float_part = rating_value % 1.0;
let star_rating = String();
for (var i = 1; i <= int_part; i++){
star_rating += '<i class="fas fa-star text-warning"></i>';
}
if (float_part >= 0.29){ // sometimes this is rounded to 0.29999999, so no half-full star would be created
star_rating += '<i class="fas fa-star-half-alt text-warning"></i>';
} else {
star_rating += '<i class="far fa-star text-warning"></i>';
}
var empty_stars = 5 - i;
for (var k = 1; k <= empty_stars; k++){
star_rating += '<i class="far fa-star text-warning"></i>';
}
return star_rating
};
Note, that you have to load the script in your html
, towards the very bottom, before the </body>
add a tag, where you link to the .js
file.
...
<script src="{{url_for('static', filename='js/misc.js')}}"></script>
</body>
Usage of star rating
Wherever you need a generated star rating in html
, you can use this function in your JavaScript
code e.g. via generate_star_rating(4.4)
and will have this html
code created:
<i class="fas fa-star text-warning"></i>
<i class="fas fa-star text-warning"></i>
<i class="fas fa-star text-warning"></i>
<i class="fas fa-star text-warning"></i>
<i class="fas fa-star-half-alt text-warning"></i>
This is the corresponding image rendered by the browser:
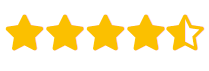